In my previous article I’ve shown how to Create calendar events using Python and if you missed that, Click here and get a basic Idea about Google Calendar API, Client Secret API JSON file and all the foundation elements of Calendar API. In this article, I’m gonna show you how to retrieve calendar events data based on a Search Query.
Alrighty! Assuming that you have all the basic idea of How the Calendar API works, I’m going to tell you a little story:
I myself and my partner Tara are psychological therapists and we have House Visits for our clients each month. We both keep track of these events in our Google Calendar. Sometimes when either of us can’t make it she passes on her shift to me and I the same. So we both used to Create our events with the title “House Visits XXX”. For an example, I have a house visit on next 24th at 2.30pm at Mrs. Michelle’s house, she’s got three kids and the husband is in prison. So my event title would be “House Visit : Mrs.Michelle”
Now you have the perfect understanding of how I’m making my living yeah? Ok cool, Let’s kick start a new Script.
from google_auth_oauthlib.flow import InstalledAppFlow
import pickle
import pprint
As usual, Declare the scope and I’ll go with auth/calendar.
PS: If you’re still not familiar with these steps, I recommend you to go to this link and start reading from the first paragraph to this exact image below. I’ve explained the steps clearly so you will not get uncomfortable with the rest of the article.
Sweet. So you’ve come to know about the Pretty Printer, Pickle, Credentials, and whatnot yeah? So this is the code I have for now:
from google_auth_oauthlib.flow import InstalledAppFlow
import pickle
import pprint
scopes = ['https://www.googleapis.com/auth/calendar']
flow = InstalledAppFlow.from_client_secrets_file("client_secret.json", scopes=scopes)
credentials = flow.run_console()
pp = pprint.PrettyPrinter(indent=4) #use pprint for nicer view
pickle.dump(credentials, open("token.pkl", "wb"))
credentials = pickle.load(open("token.pkl", "rb"))
service = build("calendar", "v3", credentials=credentials)
calendarlist = service.calendarList().list().execute()
calendar_id = calendarlist['items'][0]['id']
Now, We’re going to use Events: List for our program here. In there we have the parameter q which enable us to pass a free text search terms to find events that match these terms in any field.
query = "House Visit"
events = service.events().list(calendarId=calendar_id, q=query, singleEvents='True' ,orderBy='startTime').execute()
Store all the events[‘items’] in a list called events
events = events.get('items', [])
One more step and we’re good to finish the Chapter 😁
I want to display
- Event Name (House Visit XXX)
- Event Date
- Attendees of the Event
I’ve written this simple python for loop (nested for loop) to achieve this task
i=1
for event in events:
start = event['start'].get('dateTime')
print(i, event['summary'])
print(start)
for attendees in event['attendees']:
atte = attendees.get('displayName', 'email')
print(attendees['displayName'] + " ( " +attendees['email']+ ")" )
i += 1
print('_____________________________________')
i=1
And the output is as follows:
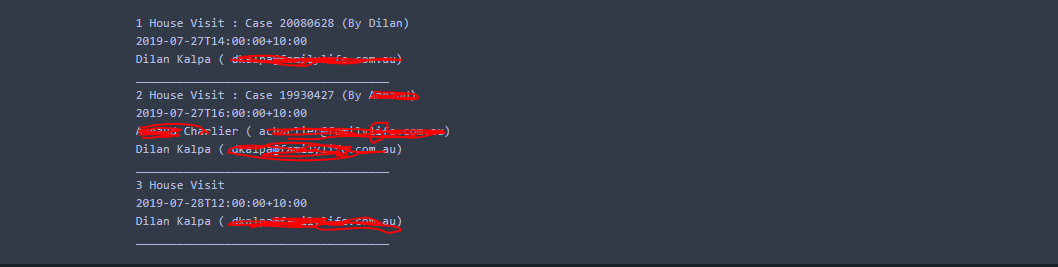
That’s how I wrap up this simple article and even though this one’s really simple and maybe some may think that this is not that much of a deal, for me and my partner this is really mean something and I thought I should share this with you guys.
Thanks a lot. Let me know if you run into any difficulties.
Furthermore, Read my article Creating Calendar events using Google Sheets data with AppScript for a more automotive experience to create Calendar Events using Google Sheet Data.
0 Comments